codegolfctober 2018
October 2018
Like last year, the codegolf team and I decided to show one codegolf thing a day for the whole month of October!
Most were new, but we also showed our favourite demos from last JS1k, JS13k and Dwitter.
Thanks to Aemkei, ETHprod, Justecorruptio, BalintCsala, Zozuar, Xen_the, Zlprp, Subzey, P01, Keithclarkcouk, Xaotic, Veubeke, Tomxor, JS1K and Dwitter for contributing to this month's awesomeness!
Day 1: Nano Unicode Slideshow
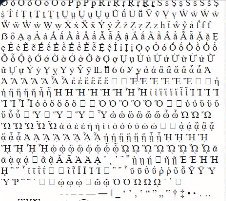
i=!setInterval(`document.body.innerHTML=""+i++`,99) // 53b
i=!setInterval`document.write(" "+i++)` // 41b, Webkit only
We reduced the "nano" demo of MiniUnicode from 54b to 53b / 41b.
The 53b version just changes how i is initialized ("i=!setInterval" instead of "i=0;setInterval")
The 41b version abuses the fact that Webkit allows to call setInterval without second parameter, and to call document.write repeatedly, which makes non-Webkit browsers freeze.
#Codegolfctober 2018, day 1:
— xem 🔵 (@MaximeEuziere) 1 octobre 2018
<script>
// Unicode slideshow in 53b:
i=!setInterval(`document.body.innerHTML="&#"+i++`,99)
// Unicode lister in 41b: (Webkit only)
i=!setInterval`document.write(" &#"+i++)`
</script>#JS pic.twitter.com/9q0UBW6U4B
Day 2: ASCII Game of Life
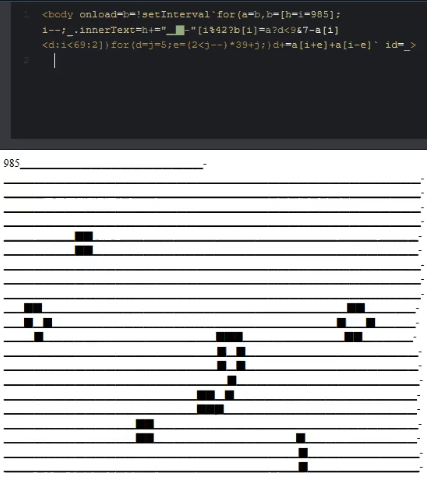
<body onload=b=!setInterval`for(a=b,b=[h=i=985];i--;_.innerText=h+="▁▉-"[i%42?b[i]=a?d<9&7-a[i]<d:i<69:2])for(d=j=5;e=(2<j--)*39+j;)d+=a[i+e]+a[i-e]` id=_>
We reduced an old Game of Life drawn in ASCII down to 165 then 159b.
The bytes saved come from a new way to compute the state of a cell based on the number of alive neighbours.
#Codegolfctober day 2
— xem 🔵 (@MaximeEuziere) 2 octobre 2018
165b ASCII Game of Life by @aemkeihttps://t.co/pohkq6JFCD
→159b with @ETHprod & @justecorruptio
<body onload=b=!setInterval`for(a=b,b=[h=i=985];i--;_.innerText=h+="▁▉-"[i%42?b[i]=a?d<9&7-a[i]<d:i<69:2])for(d=j=5;e=(2<j--)*39+j;)d+=a[i+e]+a[i-e]` id=_> pic.twitter.com/hwBUhGfzVB
Days 3 & 4: mini 2D vector library
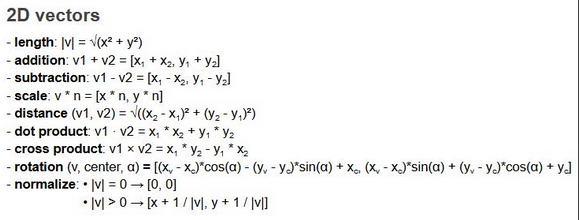
// API
//- vec2(x,y)
//- length(v)
//- add(v,w)
//- sub(v,w)
//- mul(v,n)
//- dist(v,w)
//- dotproduct(v,w)
//- crossproduct(v,w)
//- rotate(v,origin,theta)
//- normalize(v)
// Not regpacked version (264b)
V=(x,y)=>({x,y})
l=v=>d(v,v)**.5
a=(v,w)=>V(v.x+w.x,v.y+w.y)
s=(v,w)=>a(v,m(w,-1))
m=(v,n)=>V(v.x*n,v.y*n)
t=(v,w)=>l(s(v,w))
d=(v,w)=>v.x*w.x+v.y*w.y
c=(v,w)=>v.x*w.y-v.y*w.x
r=(v,o,t)=>a(o,V(c(f=s(v,o),g=V(Math.sin(t),Math.cos(t))),d(f,g)))
n=v=>m(v,1/(l(v)||1))
We had fun golfing this lib of 10 functions in 264b, by using functions inside other functions as much as possible to save bytes.
For example, the distance between two vectors is "length(sub(v,w))" instead of Math.sqrt(v.x-w.y, v.y-w.y).
And sub is also reusing add and mul instead of redoing the whole operation.
And length(v) returns the square root of dot(v, v), which corresponds to v.x² + v.y². Etc...
I'm very impressed by the solution they came up with for rotate, but I gave up trying to understand it ^^
#Codegolfctober day 3
— xem 🔵 (@MaximeEuziere) 3 octobre 2018
I'm working on a mini 2D rigid bodies physics library, and started with a full 2D vector toolkit in 264 bytes:https://t.co/PHS03AjJMR
Thanks to @BalintCsala, @justecorruptio & @ETHprod for their great help!
(Join our slack room > https://t.co/zzNoinR00N) pic.twitter.com/svULT7J59Q
// Regpackable version (236b regpacked)
v=(x,y,t)=>({x,y});
l=(x,y,t)=>d(x,x)**.5;
a=(x,y,t)=>v(x.x+y.x,x.y+y.y);
s=(x,y,t)=>a(x,m(y,-1));
m=(x,y,t)=>v(x.x*y,x.y*y);
t=(x,y,t)=>l(a(x,m(y,-1)));
d=(x,y,t)=>x.x*y.x+x.y*y.y;
c=(x,y,t)=>x.x*y.y-x.y*y.x;
r=(x,y,t)=>a(y,v(c(a(x,m(y,-1)),v(Math.sin(t),Math.cos(t))),d(a(x,m(y,-1)),v(Math.sin(t),Math.cos(t)))));
n=(x,y,t)=>m(x,1/(l(x)||1));
The next day, we optimized it to be as compressible by Regpack as possible, by repeating signatures, semicolons, and expanding some factorizations to maximize repetitions. The result fits in 236b.
#Codegolfctober day 4:
— xem 🔵 (@MaximeEuziere) 4 octobre 2018
The same 2D vector lib, optimized for Regpack (335b), then Regpacked (236b)
Made with @BalintCsala, @justecorruptio & @ETHprod <3https://t.co/lmkIlGiJQ2 pic.twitter.com/EmPvQrWHht
Day 5: Mini Music Player
with(new AudioContext)with(G=createGain())for(i in D=[...Array(26).keys()])with(createOscillator())if(D[i])connect(G),G.connect(destination),start(i*.2),frequency[v="setValueAtTime"](440*1.06**(13-D[i]),i*.2),gain[v](1,i*.2),gain.setTargetAtTime(.001,i*.2+.18,.005),stop(i*.2+.19)
A tiny music player, including the output of MiniMusic (golfed during js13kgames 2017 and 2018 and regolfed here in 262 bytes) combined with the smallest code that generates an array from 1 to N (gorfed during the day 10 of golfctober 2017, 20 bytes).
The result is a 280b script playing 2 octaves in decrescendo, while avoiding to make a "click" at the end of each note, that Oscillators do by default when we stop them abruptly.
(warning, the volume is loud by default)
#Codegolfctober day 5
— xem 🔵 (@MaximeEuziere) 5 octobre 2018
A music player in 262bhttps://t.co/5oxaEpbcON
D is a list of notes (here, 0 to 26 = 2 octaves)
The rest of the code (setValueAtTime, setTargetAtTime) prevents the browser to "click" at the end of each note
Editor: https://t.co/KeiphbXzXM
Made for #js13k
Day 6: Strange Crystals v0.140b
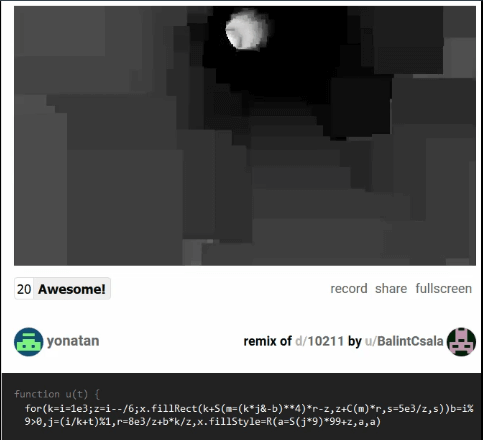
for(k=i=1e3;z=i--/6;x.fillRect(k+S(m=(k*j&-b)**4)*r-z,z+C(m)*r,s=5e3/z,s))b=i%9>0,j=(i/k+t)%1,r=8e3/z+b*k/z,x.fillStyle=R(a=S(j*9)*99+z,a,a)
@BalintCsala and @zozuar golfed a 140b demake of Strange Crystals II (winning js1k 2013 entry by @ehouais).
@zozuar wrote an amazing writeup about it here.
#Codegolfctober day 6
— xem 🔵 (@MaximeEuziere) 6 octobre 2018
The incredible dweet by @BalintCsala "strange crystals v0.140b", and its complete deconstruction by @zozuar
Dweet: https://t.co/wkEGQXJKXo
Writeup: https://t.co/0d6enkGDuC
pic.twitter.com/O2tybjY4eu
Days 7 & 8 & 11 & 16 & 30: Mini Serpinski
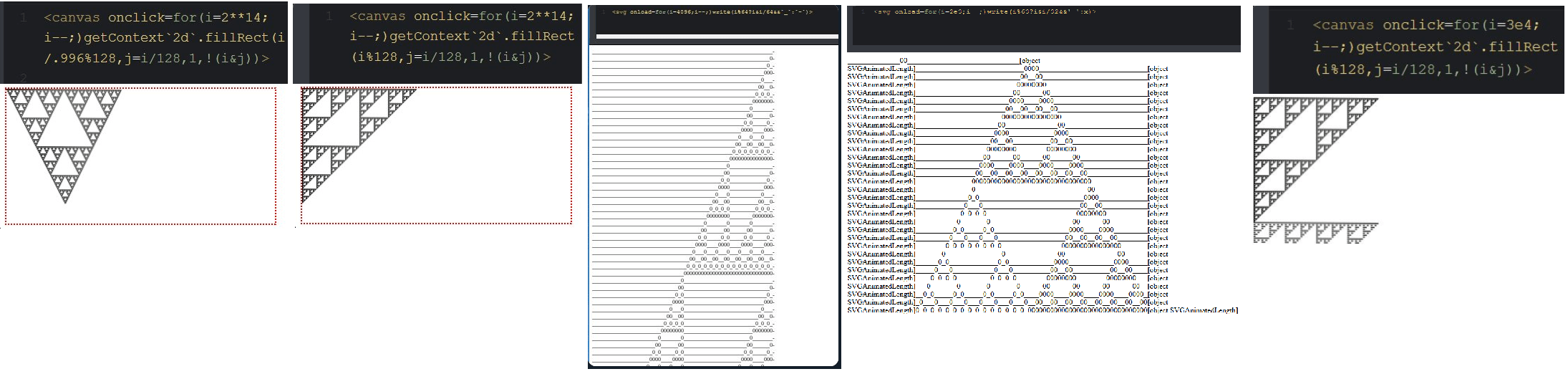
During this month, most of the time on Slask was used to golf mini Serpinski triangles on canvas and in ASCII, straight or lopsided, centered or right-aligned. Here are the different achievements of the team:
<canvas onclick=for(i=2**14;i--;)getContext`2d`.fillRect(i/.996%128,j=i/128,1,!(i&j))>
#Codegolfctober day 7
— xem 🔵 (@MaximeEuziere) 7 octobre 2018
Our 2017 Mini Serpinski Triangle was regolfed by @Xen_the, @zlprp & @justecorruptio and was reduced from 118b to 86b!
<canvas onclick=for(i=2**14;i--;)getContext`2d`.fillRect(i/.996%128,j=i/128,1,!(i&j))>
Demo (click to start): https://t.co/U4JtxIG5nH pic.twitter.com/qDlU70GEtR
<canvas onclick=for(i=2**14;i--;)getContext`2d`.fillRect(i%128,j=i/128,1,!(i&j))>
#codegolfctober day 8
— xem 🔵 (@MaximeEuziere) 8 octobre 2018
Yesterday's Mini Serpinski Triangle but "lopsided", made by @justecorruptio in 81b.
<canvas onclick=for(i=2**14;i--;)getContext`2d`.fillRect(i%128,j=i/128,1,!(i&j))>
Demo: https://t.co/ZhxxaQqw3c pic.twitter.com/sn07BxnSbt
<svg onload=for(i=4096;i--;)write(i%64?i&i/64&&`_`:`-`)>
#codegolfctober day 11
— xem 🔵 (@MaximeEuziere) 11 octobre 2018
56b ASCII Serpinski "right-aligned" by @justecorruptio, @aemkei & @keithclarkcouk
<svg onload=for(i=4096;i--;)write(i%64?i&i/64&&`_`:`-`)>
Demo: https://t.co/Tpuav4tDBh
(requires a narrow window to show line breaks at the right places) pic.twitter.com/i6446e1yFC
<svg onload=for(i=2e3;i--;)write(i%63?i&i/32&&'_':x)>
#codegolfctober day 16:
— xem 🔵 (@MaximeEuziere) 16 octobre 2018
The smallest (and weirdest) ASCII Serpinski Triangle by @aemkei, @keithclarkcouk and @justecorruptio
53 bytes!
<svg onload=for(i=2e3;i--;)write(i%63?i&i/32&&'_':x)>
Demo: https://t.co/CKhOt9x9LH
(needs a window < ~1130px) pic.twitter.com/omAs3KrJqj
<canvas onclick=for(i=3e4;i--;)getContext`2d`.fillRect(i%128,j=i/128,1,!(i&j))>
#codegolfctober day 30@Xen_the reduced the lopsided mini Serpinski triangle (cf. day 8) to 79b!
— xem 🔵 (@MaximeEuziere) 30 octobre 2018
<canvas onclick=for(i=3e4;i--;)getContext`2d`.fillRect(i%128,j=i/128,1,!(i&j))>
Demo: https://t.co/MQQYE8UPOD
(click canvas to start) pic.twitter.com/MxA2iiD5BQ
Days 9 & 10 & 12 & 13: MiniCalculator
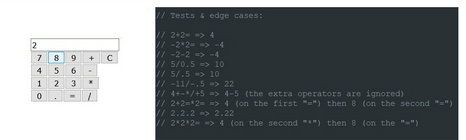
A wild codegolf project appeared on day 9, based on an idea prototyped by @ETHprod: a mini calculator!
During the following week, we golfed it in two different versions: full-featured and minimalist.
It was pretty fun to golf the generation of the UI (input, buttons), while implementing each button's features, while precisely matching the behavior of a real calculator in every possible edge case. Boy, those things are weird, but our code is even weirder!
<!-- Full-featured calc -->
<body onload='for(s of"C/=.0 *321 -654 +987 ")i.outerHTML+=s<"!"?"<p>":`<button onclick=F("${s}")>`+s;F=b=>i.value=z=b>"B"?(c=d=o="",a=r=p=0):1+b-0?c+=b-.1?b:d?"":d=b:(q=b<"=",p?r=c:a=c||a,r=q?p&&r:r||a,a=o&&r?z=eval(a+o+s+r):a,o=q?b:o,p=q,d=c="",q?z:a);F`C`'><input id=i>
#codegolfctober day 9
— xem 🔵 (@MaximeEuziere) 9 octobre 2018
A 252b calculator in HTML/JS inspired by @ETHprod
Demo: https://t.co/vhD4kZIP7r
I summon @Xen_the @subzey @aemkei @p01 and @justecorruptio to bring that below 200b :p pic.twitter.com/WX03uCfxKP
#codegolfctober day 10@justecorruptio successfully golfed yesterday's calculaton in 197b!
— xem 🔵 (@MaximeEuziere) 10 octobre 2018
In the meantime, I made a 770b calculator in HTML/JS but this time it supports every weird edge case!
Demo: https://t.co/0BpY9ToZWx@Xen_the @subzey @aemkei @p01, let's golf it? pic.twitter.com/2KF1HS6pT0
#codegolfctober day 12
— xem 🔵 (@MaximeEuziere) 12 octobre 2018
272b full-featured calculator (the one from day 10, but rewritten and corrected by @justecorruptio)
Source: https://t.co/UJwhes0tJr
Demo: https://t.co/KlYqDay3JO pic.twitter.com/DrFn2jbj2A
<input id=i><script>o='';for(s of"C/=.0!*321!-654 +987!")i.outerHTML+=s<"#"?"<p>":`<button onclick="o=i.value=${s=="="?`eval(o)`:s>"B"?`''`:`o+'${s}'`}">`+s</script>
#codegolfctober day 13:
— xem 🔵 (@MaximeEuziere) 13 octobre 2018
165b super-basic calculator (HTML+JS)
Demo: https://t.co/XDIDI4xd8r
Source code:
<input id=i><script>o='';for(s of"C/=.0!*321!-654 +987!")i.outerHTML+=s<"#"?"<p>":`<button onclick="o=i.value=${s=="="?`eval(o)`:s>"B"?`''`:`o+'${s}'`}">`+s</script> pic.twitter.com/MVrGt05Ad4
Days 14, 15: MiniSweeper
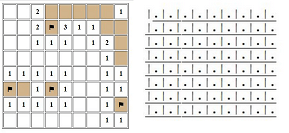
My oldest codegolfed game, made in 497b with Subzey back in 2013, lost 11 bytes after I used ES6 and removed old browsers hacks.
<table border id=t><script>b=[v=[]];f=[l=g=0];n=[];r=d=>{for(M=!l;M;l=g)for(a=M=H="";s>++a;)for(j=s,H+="<tr>";~--j;H+=`<th width=25 height=30 onclick=b[i=${I}]?g--:v[i]=1;r() oncontextmenu=for(f[${I}]^=g=1,a=0;a<s*s;)g&=b[a]^!f[a++];return!!r() ${(v[I]|g||"bgcolor=tan")}>`+(f[I]?"⚑":b[I]&g?"💣":v[I]|g&&n[I]||""))for(I=a*s+j,T=d?b[I]=.1>Math.random():0,x=2;~x--;)for(y=2;~y--;~B&&B<s?(n[C]=~~n[C]+T)||v[I]|b[I]|!v[C]?0:v[I]=M=1:0)B=j+y,C=(a+x)*s+B;t.innerHTML=H};r(s=9)</script>
#codegolfctober day 14:
— xem 🔵 (@MaximeEuziere) 14 octobre 2018
My first codegolf project, made with @subzey back in 2013,
rebooted with more ES6 and less IE hacks: 486 bytes!
I'm sure we can enhance it.
Demo: https://t.co/tIzxsVp4Ry
Commented source code: https://t.co/zz15sVxxYm pic.twitter.com/cA8M0pyEeY
Then Aemkei joined the party and brought it down to 349b while converting it to ASCII art, which means 148 bytes saved!
<pre id=t><script>b=[v=[]];l=g=0;n=[];r=d=>{for(M=!l;M;l=g)for(a=M=H="";s>++a;)for(j=s,H+=`
`;~--j;t.innerHTML=H+=`|<u onclick=b[i=${I}]?g--:v[i]=1;r()>`+(b[I]&g?"#":v[I]|g&&n[I]||(v[I]|g?" ":'.')))for(I=a*s+j,T=d?b[I]=.1>Math.random():0,x=2;~x--;)for(y=2;~y--;~B&&B<s?(n[C]=~~n[C]+T)||v[I]|b[I]|!v[C]?0:v[I]=M=1:0)B=j+y,C=(a+x)*s+B};r(s=9)</script>
#codegolfctober day 15:
— xem 🔵 (@MaximeEuziere) 15 octobre 2018
MiniSweeper demake in ASCII and in 349 bytes by @aemkei !
Demo: https://t.co/E0cBy0yexm
(play with left click only, this version doesn't have flags) pic.twitter.com/I8NBMfc93H
Day 17: Mini Keyboard

The smallest keyboard state manager, in 46b, adapted from this article and previous golfed projects.
k={};onkeydown=onkeyup=e=>k[e.which]=e.type[5]
#codegolfctober day 17:
— xem 🔵 (@MaximeEuziere) 17 octobre 2018
A keydown/keyup state manager in 46 bytes (useable in a game loop)
k={};onkeydown=onkeyup=e=>k[e.which]=e.type[5]
- Choose a keyCode N.
- if(k[N]) => key is pressed
- if(!k[N]) => key is released
Demo: https://t.co/Hrfpn1c1YM pic.twitter.com/arK781cSu7
Day 18: Mini Simon
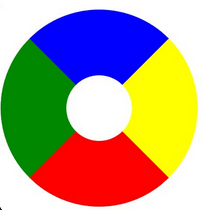
I regolfed our old Simon game and saved 53 bytes by removing old browser hacks and applying the new sound generation techniques from day 5, but even more simplified, because only four different beeps were required.
The frequencies of the buttons k=0 to k=3 are computed with "frequency.value=99+k*99". The first 99 makes the sound "0" audible, and the second 99 makes the sounds different enough from each other.
<body onload="a=(k,q)=>{z=['00F','FF0','F00','080'];if(~k){with(new AudioContext)with(createOscillator())start(0,connect(destination),frequency.value=99+k*99)+stop(.2,s(a,250,-1));z[(3+k)%4]=111};p.style.borderColor='#'+z.join`#`;if(q)k^f[j]?p.outerHTML=':(':++j^g||h()};(h=e=>{for(i=g=f.push(new Date%4);j=i;s(a,500*i--,f[i]))s=setTimeout})(f=[])"onkeyup=a(event.keyCode-37,1) style="width:9em;height:9em;border-radius:50%;border:9em solid"id=p>
#codegolf day 18:
— xem 🔵 (@MaximeEuziere) 18 octobre 2018
A Simon game with sound in 446b
(the old version was 499b)
Demo: https://t.co/09EnpgFqI3
(click on the wheel then play with arrow keys) pic.twitter.com/AI4JopBygY
Day 19: Print all the chars that the program doesn't contain
Our first attempt back in 2013 took 88 bytes, this tiime it's down to 74b, due to a completely different approach involving ES6 and very hacky things.
eval(v="for(i=32;i<127;)v.includes(e=String.fromCharCode(i++))||alert(e)")
#codegolfctober day 19
— xem 🔵 (@MaximeEuziere) 19 octobre 2018
Old CGSE challenge
"a program that outputs all the ASCII chars that it doesn't contain"
eval(v="for(i=32;i<127;)v.includes(e=String.fromCharCode(i++))||alert(e)")
// 74b, alerts the chars !"#$%&'*,-/045689:>?@ ABDEFGHIJKLMNOPQRTUVWXYZ[\]^_`bjkpqwxyz{}~
Day 20: Char generation
We explored many ways to generate a char from an integer, to improve day 19's challenge. As far as we know, there are 4 solutions below 30 bytes, but none of them beats String.fromCharCode(i)...
#codegolfctober day 20
— xem 🔵 (@MaximeEuziere) 20 octobre 2018
How to generate the i'th ASCII char in JS?
var i=33;
eval(`'\\x${i.toString(16)}'`) // 30b
eval(`'\\${i.toString(8)}'`) // 28b
String.fromCodePoint(i) // 23b
String.fromCharCode(i) // 22b
Could the 28b version be used to improve yesterday's challenge? 🤔
Day 21: Space dweets
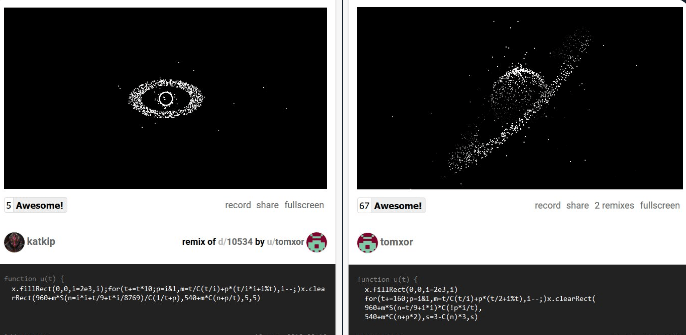
Promoting some great dweets of Tomxor:
Black Hole: DEMO
Saturn: DEMO
#codegolfctober day 21
— xem 🔵 (@MaximeEuziere) 21 octobre 2018
Here are my favourite "space" dweets of October, by Tomxor
(I didn't contribute, but they deserves more views!)
Black Hole: https://t.co/1Sq6ClDDTQ
Saturn: https://t.co/AQ0GQg536T pic.twitter.com/cjI8P5C259
Day 22: Mini Split Screen
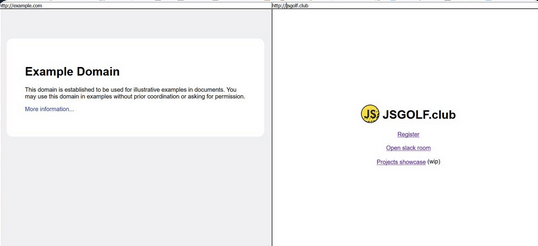
I had done this little split screen browser app last year, using 2, 3, 4 or 6 iframes to avoid having many browser windows in parallel on the same screen, and preventing one of the iframes to change the URL of the parent window.
Now it's golfed in 225b, and with a lot of weird CSS to make it look nice on any screen size!
<body onbeforeunload=return!1><input oninput=c.src=value><input oninput=d.src=value><iframe id=c></iframe><iframe id=d></iframe><style>*{margin:0;padding:0;box-sizing:border-box}body *{width:50%}#c,#d{height:calc(100vh - 20px
#codegolfctober day 22
— xem 🔵 (@MaximeEuziere) 22 octobre 2018
225b split screen browser
Source: https://t.co/CNGXXeANWJ
Demo: https://t.co/JmfhGG2LZU
(also exists in x3, x4 and x6) pic.twitter.com/02VxSSiWZe
Day 23: Mini Sound Player
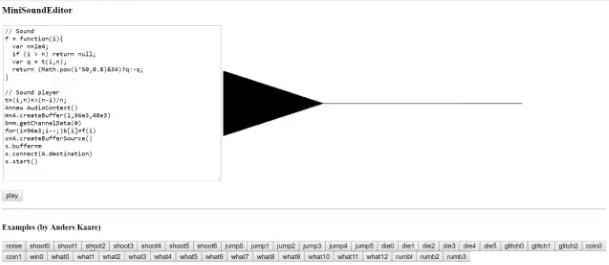
I took the sound player (and sound collection) that Anders Kaare made for our js13k16 and 17 entries, and gathered them in an app that's able to edit, export, play and even draw each sound with a 2-second limit. I also golfed the player in 176b and the result is below. It needs a custom function f(i) that gives the value of each sample.
t=(i,n)=>(n-i)/n;
A=new AudioContext()
m=A.createBuffer(1,96e3,48e3)
b=m.getChannelData(0)
for(i=96e3;i--;)b[i]=f(i)
s=A.createBufferSource()
s.buffer=m
s.connect(A.destination)
s.start()
#golfctober day 23
— xem 🔵 (@MaximeEuziere) 23 octobre 2018
176b sound player
A=new AudioContext()
m=A.createBuffer(1,96e3,48e3)
b=m.getChannelData(0)
for(i=96e3;i--;)b[i]=f(i)
s=A.createBufferSource()
s.buffer=m
s.connect(A.destination)
s.start()
f() generates the buffer's samples
More info: https://t.co/KWj5OHboHZ
Day 24: Mega Million Picker
The Mega Million lottery happened that month in the USA and people on Twitter tried to make a Mega Million number picker, with specific rules (Picks 5 unique ordered numbers between 1 and 70, then 1 number between 1 and 25).
We joined them and made this version in 88b and a lot of rarely used before ES6 features.
[z,...a]=Array(71).keys(),[...(f=_=>a[5]?f(a.splice(Math.random(z++)*99,1)):a)(),z%25+1]
#codegolfctober day 24
— xem 🔵 (@MaximeEuziere) 24 octobre 2018
88b Mega Million picking generator
[z,...a]=Array(71).keys(),[...(f=_=>a[5]?f(a.splice(Math.random(z++)*99,1)):a)(),z%25+1]
(Picks 5 unique ordered numbers between 1 and 70, then 1 number between 1 and 25.)
Golfed by @subzey @superhoge @kchplr @p01 & me
Day 25: Mini LOSSST
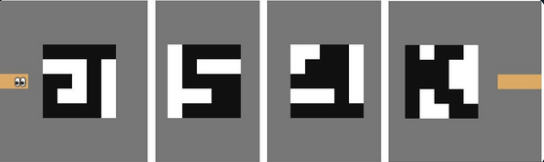
A little throwback to our 1kb LOSSST demake (a.k.a. SN1KE) made during JS1K 2017.
#codegolfctober day 25
— xem 🔵 (@MaximeEuziere) 26 octobre 2018
In case you missed it, here's a demake of my #js13k puzzle game LOSSST, including 55 levels, golfed in 1kb with @Xen_the, @Xaotic, @justecorruptio, @subzey, @p01 and @veubeke during js1k 2018
Play: https://t.co/XgtAinNfWz
Making-of https://t.co/8AS8xeikuq pic.twitter.com/kz6mdRU53Y
Days 26 and 27: Mini Fourier
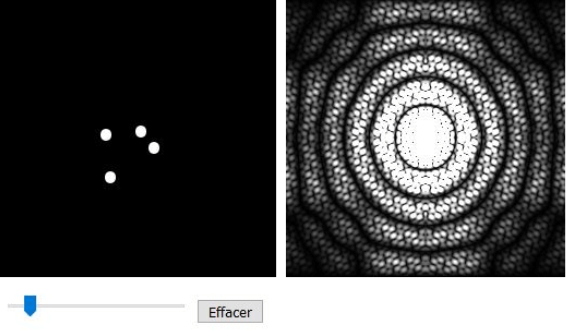
Another js1k 2017 throwback with this instant 2D FFT tracer.
#codegolfctober day 26
— xem 🔵 (@MaximeEuziere) 26 octobre 2018
Another js1k 2018 entry: an interactive 2D FFT tracer golfed w/ @subzey @BalintCsala @justecorruptio
play: https://t.co/16wSipTATG
making-of: https://t.co/8AS8xeikuq
Detailed source code, including the smallest JS FFT ever writtenhttps://t.co/FMiW1fvW6u pic.twitter.com/5DfglgvojA
The next day, we isolated and golfed the 1D FFT function from this js1k entry, in 312b.
F=(e,f)=>{M=Math;n=e.length;for(i=0;i<n;i++){for(j=0,h=i,k=n;k>>=1;h>>=1)j=j<<1|h&1;j>i&&([e[j],e[i],f[j],f[i]]=[e[i],e[j],f[i],f[j]])}for(h=1;2*h<=n;h*=2)for(i=0;i<n;i+=2*h)for(j=i;j<i+h;j++)l=M.cos(k=M.PI*(j-i)/h),m=M.sin(k),o=e[j+h]*l+f[j+h]*m,p=-e[j+h]*m+f[j+h]*l,e[j+h]=e[j]-o,f[j+h]=f[j]-p,e[j]+=o,f[j]+=p}
#codegolfctober day 27
— xem 🔵 (@MaximeEuziere) 27 octobre 2018
Here's yesterday's JS FFT golfed in 312b
Demo: https://t.co/vKDitiLawZ
(leaks vars M,h,i,j,k,l,m,n,o,p)
Based on this 359b FFT by @antimatter15:https://t.co/VCNlgbJRlS
Beats this "140b FFT" that requires ~550b of bootstrap code:https://t.co/MoEY2SzmZG pic.twitter.com/7i2MXcWgSS
Days 28 & 29: Epic Cycles
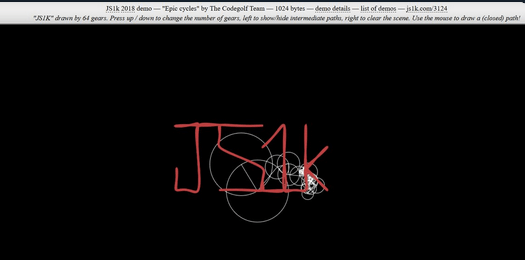
Final throwback to JS1K 2017 with our 1kb epicycloid tracer
#codegolfctober day 28
— xem 🔵 (@MaximeEuziere) 28 octobre 2018
A 1KB interactive epicycloid editing/tracing app golfed with @BalintCsala during last JS1K
Play: https://t.co/YGjUpfrP1I
Making-of: https://t.co/8AS8xeikuq
Detailed source code: https://t.co/0JkD9eB4M7 pic.twitter.com/KepkiC9DqU
The next day; we isolated the 1D DFT function from this entry (simpler than a FFT, but slower), and golfed it in 161b
D=n=>{for(i in _=[],M=Math,n)for(k in _[i]=[0,0],n)b=M.cos(a=-2*k*M.PI*i/n.length),c=M.sin(a),_[i][0]+=n[k][0]*b-n[k][1]*c,_[i][1]+=n[k][1]*b+n[k][0]*c;return _}
#codegolfctober day 29
— xem 🔵 (@MaximeEuziere) 29 octobre 2018
To save bytes, EpicCycles wasn't using FFT but DFT
Here's the smallest (161b) DFT in JS golfed w/ @BalintCsala:
(it works on a 2D array and leaks vars _,a,b,c)
Demo: https://t.co/xm4AMF3J6o
Tweet: https://t.co/z1k8zOzSoW
Based on: https://t.co/tDzh46b0TW pic.twitter.com/mSgub2Tisf
Day 31: Mini Peach Castle
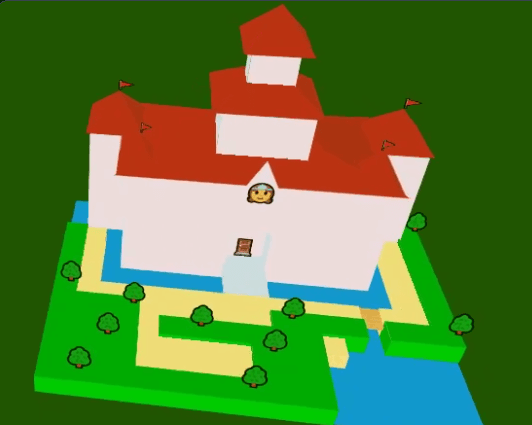
The first sneak peek of my WIP entry for JS1K 2019: Mario 64's Peach Castle, as complete as possible, in 1kb, and rendered with HTML and CSS3D. More details soon!
#codegolfctober day 31
— xem 🔵 (@MaximeEuziere) 31 octobre 2018
A super hacky prototype of JS1K 2019 entry containing Peach Castle (from Mario 64) rendered in CSS3D and emoji, in 1026 bytes (minified and regpacked)...
and 3330 bytes before regpack.
Demo: https://t.co/iOkewmbPG3
(optimized for Windows + Chrome) pic.twitter.com/aKOzuxnQS3